MST
mst : minimum spanning tree(최소 신장 트리)
최소 비용 신장 트리
최소 신장 트리란 그래프 내에서 모든 간선을 연결하는 최소 비용의 트리를 의미한다.
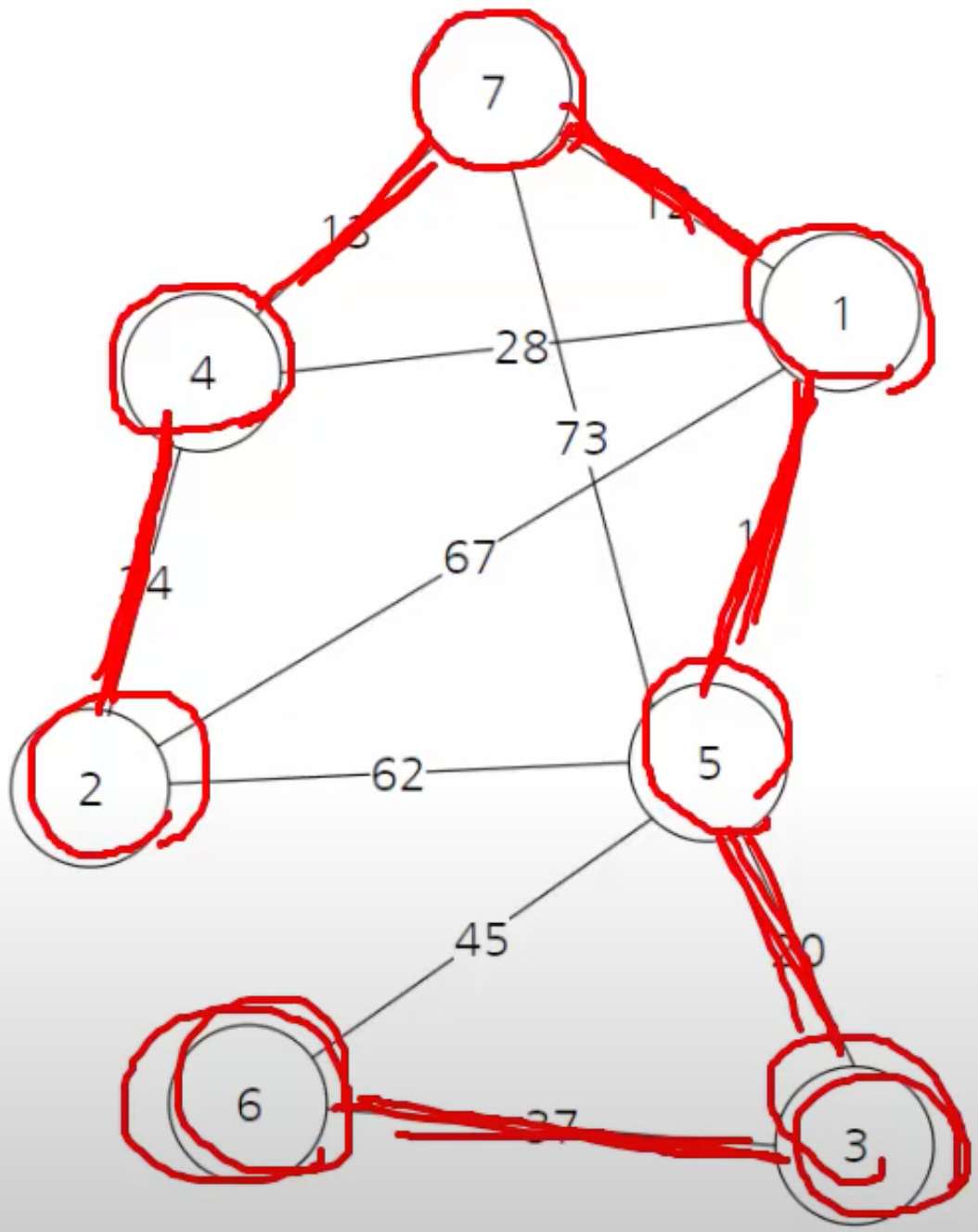
최소 신장 트리는 Cycle
이 발생하지 않아야하며, N-1
개의 간선을 가지고 있어야 한다.
Kruskal Algorithm
간단하게 설명하자면,
- parent가 더 작은 쪽이 부모가 되도록 한다.
- parent가 같다면 cycle이 발생하므로 무시한다.
- parent가 다르다면 union을 통해 합친다.
- 모든 간선을 확인할 때까지 1~3을 반복한다.
import sys
def find_parent(parent, a):
if parent[a] != a:
parent[a] = find_parent(parent, parent[a])
return parent[a]
def union_parent(private_parent, a, b):
a = find_parent(private_parent, a)
b = find_parent(private_parent, b)
if a < b:
private_parent[b] = a
else:
private_parent[a] = b
input = sys.stdin.readline
v, e = map(int, input().split())
parent = [0] * (v + 1)
for i in range(1, v + 1):
parent[i] = i
edges = []
result = 0
for _ in range(e):
a, b, cost = map(int, input().split())
edges.append((a, b, cost))
# cost를 기준으로 정렬
edges.sort(key=lambda x: x[2])
for a, b, cost in edges:
if find_parent(parent, a) != find_parent(parent, b):
union_parent(parent, a, b)
result += cost
print(result)
Reference
Prim Algorithm
import heapq
import sys
sys.setrecursionlimit(10 ** 6)
input = sys.stdin.readline
n, m = map(int, input().split()) # 노드 수, 간선 수
graph = [[] for _ in range(n + 1)]
visited = [0] * (n + 1) # 노드의 방문 정보 초기화
# 무방향 그래프 생성
for i in range(m): # 간성 정보 입력 받기
u, v, weight = map(int, input().split())
graph[u].append([weight, u, v])
graph[v].append([weight, v, u])
# 프림 알고리즘
def prim(graph, start_node):
visited[start_node] = 1 # 방문 갱신
candidate = graph[start_node] # 인접 간선 추출
heapq.heapify(candidate) # 우선순위 큐 생성
mst = [] # mst
total_weight = 0 # 전체 가중치
while candidate:
weight, u, v = heapq.heappop(candidate) # 가중치가 가장 적은 간선 추출
if visited[v] == 0: # 방문하지 않았다면
visited[v] = 1 # 방문 갱신
mst.append((u, v)) # mst 삽입
total_weight += weight # 전체 가중치 갱신
for edge in graph[v]: # 다음 인접 간선 탐색
if visited[edge[2]] == 0: # 방문한 노드가 아니라면, (순환 방지)
heapq.heappush(candidate, edge) # 우선순위 큐에 edge 삽입
return total_weight
print(prim(graph, 1))
Reference
https://deep-learning-study.tistory.com/595#google_vignette (opens in a new tab)